mirror of
https://github.com/chylex/Nextcloud-Desktop.git
synced 2024-11-14 07:42:46 +01:00
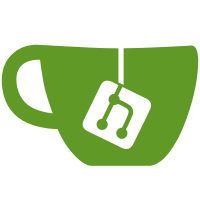
Now that csync builds as C++, this will avoid having to implement functionalities needed by csync mandatorily in csync itself. This library is built as part of libocsync and symbols exported through it. This requires a relicense of Utility as LGPL. All classes moved into this library from src/libsync will need to be relicensed as well.
46 lines
1.2 KiB
C++
46 lines
1.2 KiB
C++
/*
|
|
* This software is in the public domain, furnished "as is", without technical
|
|
* support, and with no warranty, express or implied, as to its usefulness for
|
|
* any purpose.
|
|
*
|
|
*/
|
|
|
|
#include <QtTest>
|
|
|
|
#include "common/utility.h"
|
|
#include "folder.h"
|
|
|
|
using namespace OCC;
|
|
|
|
class TestFolder: public QObject
|
|
{
|
|
Q_OBJECT
|
|
private slots:
|
|
void testFolder()
|
|
{
|
|
QFETCH(QString, folder);
|
|
QFETCH(QString, expectedFolder);
|
|
Folder *f = new Folder("alias", folder, "http://foo.bar.net");
|
|
QCOMPARE(f->path(), expectedFolder);
|
|
delete f;
|
|
}
|
|
|
|
void testFolder_data()
|
|
{
|
|
QTest::addColumn<QString>("folder");
|
|
QTest::addColumn<QString>("expectedFolder");
|
|
|
|
QTest::newRow("unixcase") << "/foo/bar" << "/foo/bar";
|
|
QTest::newRow("doubleslash") << "/foo//bar" << "/foo/bar";
|
|
QTest::newRow("tripleslash") << "/foo///bar" << "/foo/bar";
|
|
QTest::newRow("mixedslash") << "/foo/\\bar" << "/foo/bar";
|
|
QTest::newRow("windowsfwslash") << "C:/foo/bar" << "C:/foo/bar";
|
|
QTest::newRow("windowsbwslash") << "C:\\foo" << "C:/foo";
|
|
QTest::newRow("windowsbwslash2") << "C:\\foo\\bar" << "C:/foo/bar";
|
|
}
|
|
|
|
};
|
|
|
|
QTEST_APPLESS_MAIN(TestFolder)
|
|
#include "testfolder.moc"
|