## PR Type
What kind of change does this PR introduce?
- Feature
<!-- - Code style update (formatting) -->
<!-- - Refactoring (no functional changes, no api changes) -->
<!-- - Build or CI related changes -->
<!-- - Documentation content changes -->
<!-- - Sample app changes -->
<!-- - Other... Please describe: -->
## What is the current behavior?
<!-- Please describe the current behavior that you are modifying, or link to a relevant issue. -->
There is currently no way to get the underlying `T[]` array from a `MemoryOwner<T>` or `SpanOwner<T>` instance without going through some hoops that are very inconvenient (and which are only possible for `MemoryOwner<T>`). Being able to use the array directly is necessary when working with some older APIs that don't offer a `Span<T>` or `Memory<T>` overload.
## What is the new behavior?
<!-- Describe how was this issue resolved or changed? -->
This PR introduces a new `DangerousGetArray` method that mirrors the `MemoryMarshal.TryGetArray` method and works on `MemoryOwner<T>` and `SpanOwner<T>` instances. I've removed the try pattern since here the types guarantee that the underlying memory store will always be an array. The methods are called `Dangerous___` because using the array is potentially dangerous in case a user keeps the array after disposing the original owner, as it means that that array might've been rented to some other consumer, so using it could lead to unexpected behavior. The methods are not inherently dangerous per se.
## API surface
```csharp
namespace Microsoft.Toolkit.HighPerformance.Buffers
{
public sealed class MemoryOwner<T>
{
public ArraySegment<T> DangerousGetArray();
}
public readonly ref struct SpanOwner<T>
{
public ArraySegment<T> DangerousGetArray();
}
}
```
## Example usage
Suppose we have a `Person` class with `string Name`, `string Surname` and `int Age` properties, and we want to calculate an MD5 hash with the current state of the class. This was originally asked by a user in the C# Discord server ([here](https://discordapp.com/channels/143867839282020352/312132327348240384/766694351383560205)).
```csharp
public static string GetMD5Hash(Person person)
{
using var buffer = new ArrayPoolBufferWriter<byte>();
buffer.Write<char>(person.Name);
buffer.Write<char>(person.Surname);
buffer.Write(person.Age);
using SpanOwner<byte> hash = SpanOwner<byte>.Allocate(16);
using var md5 = MD5.Create();
md5.TryComputeHash(buffer.WrittenSpan, hash.Span, out _);
return BitConverter.ToString(hash.DangerousGetArray().Array!, 0, 16);
}
```
You can see how here we can leverage the new `DangerousGetArray` API to get the underlying array to use with the `BitConverter.ToString` API, which doesn't have an overload accepting a `ReadOnlySpan<byte>`. The same goes for many other existing APIs that only accept an array as input data instead of the new memory APIs.
## PR Checklist
Please check if your PR fulfills the following requirements:
- [X] Tested code with current [supported SDKs](../readme.md#supported)
- [ ] ~~Pull Request has been submitted to the documentation repository [instructions](..\contributing.md#docs). Link: <!-- docs PR link -->~~
- [ ] ~~Sample in sample app has been added / updated (for bug fixes / features)~~
- [ ] ~~Icon has been created (if new sample) following the [Thumbnail Style Guide and templates](https://github.com/windows-toolkit/WindowsCommunityToolkit-design-assets)~~
- [X] Tests for the changes have been added (for bug fixes / features) (if applicable)
- [X] Header has been added to all new source files (run *build/UpdateHeaders.bat*)
- [X] Contains **NO** breaking changes
## PR Type
What kind of change does this PR introduce?
<!-- Please uncomment one or more that apply to this PR. -->
- Optimization
<!-- - Bugfix -->
<!-- - Feature -->
<!-- - Code style update (formatting) -->
<!-- - Refactoring (no functional changes, no api changes) -->
<!-- - Build or CI related changes -->
<!-- - Documentation content changes -->
<!-- - Sample app changes -->
<!-- - Other... Please describe: -->
## What is the current behavior?
<!-- Please describe the current behavior that you are modifying, or link to a relevant issue. -->
The `ArrayPoolBufferWriter<T>` uses the given `ArrayPool<T>` instance to resize its internal buffer when needed, which only works as expected when the array itself is small. The issue is that the `ArrayPool<T>.Shared` instance has an internal threshold set to `1024 * 1024`, over which it just allocates new arrays every time to avoid keeping very large arrays alive for a long time. That is perfectly fine, except for one little detail: once you get past that threshold, `ArrayPool<T>.Shared` **stops rounding up the requested size**. It instead returns an array with `new[]` of **exactly the requested size**, which absolutely kills the performance when used in a writer type like `ArrayPoolBufferWriter<T>`: this means that as soon as we get past tha threshold, we'll basically end up resizing the whole array for every single new write operation, no matter how large it is. That's super bad for performance and memory usage 🥺
## What is the new behavior?
<!-- Describe how was this issue resolved or changed? -->
The solution for this is pretty simple, this PR includes a simple check for the requested size, and if that's over `1024 * 1024` it just rounds that up to the closest power of 2, so that the array size will effectively just keep being multiplied by 2 every time. This has a **huge** performance impact when eg. trying to use the `ArrayPoolBufferWriter<T>` class to write a 10MB buffer, 8KB at a time:
| Method | Mean | Error | StdDev | Ratio | Gen 0 | Gen 1 | Gen 2 | Allocated |
|-------------------------- |-------------:|-----------:|-----------:|------:|------------:|------------:|------------:|-----------:|
| Before | 1,380.236 ms | 19.9806 ms | 17.7122 ms | 1.000 | 355000.0000 | 355000.0000 | 355000.0000 | 5754.44 MB |
| After | **8.820 ms** | 0.1757 ms | 0.4838 ms | **0.006** | 640.6250 | 640.6250 | 640.6250 | **30 MB** |
In this simple benchmark alone, the updated version is **156x** faster and uses **190x less memory** 😄
Of course, results will vary a lot on the specific workload, but you can imagine the impact being even more dramatic when working with larger buffers, or with less items being written at any given time. With this change in general, users will not have to worry about the size of the data being written, and the class will automatically use the right approach in all cases.
## PR Checklist
Please check if your PR fulfills the following requirements:
- [X] Tested code with current [supported SDKs](../readme.md#supported)
- [ ] ~~Pull Request has been submitted to the documentation repository [instructions](..\contributing.md#docs). Link: <!-- docs PR link -->~~
- [ ] ~~Sample in sample app has been added / updated (for bug fixes / features)~~
- [ ] ~~Icon has been created (if new sample) following the [Thumbnail Style Guide and templates](https://github.com/windows-toolkit/WindowsCommunityToolkit-design-assets)~~
- [X] Tests for the changes have been added (for bug fixes / features) (if applicable)
- [X] Header has been added to all new source files (run *build/UpdateHeaders.bat*)
- [X] Contains **NO** breaking changes
## Follow up to #3428
<!-- Add the relevant issue number after the "#" mentioned above (for ex: Fixes#1234) which will automatically close the issue once the PR is merged. -->
This PR is for tracking all changes/fixes/improvements to the `Microsoft.Toolkit.Mvvm` package following the Preview 3.
<!-- Add a brief overview here of the feature/bug & fix. -->
## PR Type
What kind of change does this PR introduce?
<!-- Please uncomment one or more that apply to this PR. -->
- Feature
- Improvements
<!-- - Code style update (formatting) -->
<!-- - Refactoring (no functional changes, no api changes) -->
<!-- - Build or CI related changes -->
<!-- - Documentation content changes -->
<!-- - Sample app changes -->
<!-- - Other... Please describe: -->
## Overview
This PR is used to track and implement new features and tweaks for the `Microsoft.Toolkit.Mvvm` package.
See the linked issue for more info, and for a full list of changes included in this PR.
## PR Checklist
Please check if your PR fulfills the following requirements:
- [X] Tested code with current [supported SDKs](../readme.md#supported)
- [ ] ~~Pull Request has been submitted to the documentation repository [instructions](..\contributing.md#docs). Link: <!-- docs PR link -->~~
- [ ] ~~Sample in sample app has been added / updated (for bug fixes / features)~~
- [ ] ~~Icon has been created (if new sample) following the [Thumbnail Style Guide and templates](https://github.com/windows-toolkit/WindowsCommunityToolkit-design-assets)~~
- [X] Tests for the changes have been added (for bug fixes / features) (if applicable)
- [X] Header has been added to all new source files (run *build/UpdateHeaders.bat*)
- [X] Contains **NO** breaking changes
## Close#3509
<!-- Add the relevant issue number after the "#" mentioned above (for ex: Fixes#1234) which will automatically close the issue once the PR is merged. -->
<!-- Add a brief overview here of the feature/bug & fix. -->
## PR Type
What kind of change does this PR introduce?
<!-- Please uncomment one or more that apply to this PR. -->
- Refactoring (no functional changes, no api changes)
<!-- - Build or CI related changes -->
<!-- - Documentation content changes -->
<!-- - Sample app changes -->
<!-- - Other... Please describe: -->
## What is the current behavior?
<!-- Please describe the current behavior that you are modifying, or link to a relevant issue. -->
There are a number of usages of `Unsafe.AsRef<T>(null)` and similar that are verbose.
## What is the new behavior?
<!-- Describe how was this issue resolved or changed? -->
These occurrences are replaced using the new `Unsafe.NullRef<T>()` and `Unsafe.IsNullRef<T>(...)` APIs.
## Notes
Leaving this as draft as the new `System.Runtime.CompilerServices.Unsafe` package is still in release candidate.
## PR Checklist
Please check if your PR fulfills the following requirements:
- [X] Tested code with current [supported SDKs](../readme.md#supported)
- [ ] ~~Pull Request has been submitted to the documentation repository [instructions](..\contributing.md#docs). Link: <!-- docs PR link -->~~
- [ ] ~~Sample in sample app has been added / updated (for bug fixes / features)~~
- [ ] ~~Icon has been created (if new sample) following the [Thumbnail Style Guide and templates](https://github.com/windows-toolkit/WindowsCommunityToolkit-design-assets)~~
- [X] Tests for the changes have been added (for bug fixes / features) (if applicable)
- [X] Header has been added to all new source files (run *build/UpdateHeaders.bat*)
- [X] Contains **NO** breaking changes
# Add ColorPickerButton to the Toolkit
Closes#3363
## PR Type
What kind of change does this PR introduce?
Feature
Documentation content changes
Sample app changes
## What is the current behavior?
The toolkit, and WinUI/UWP, has no flyout ColorPicker. The color picker that currently exists is a large canvas-type control (that is incorrectly named). This existing control has lots of usability concerns discussed in #3363 and the WinUI repository.
## What is the new behavior?
A brand-new control is added to allow selecting and editing a color within a flyout. The selected color is always visible in the content area of a drop-down button as a preview.
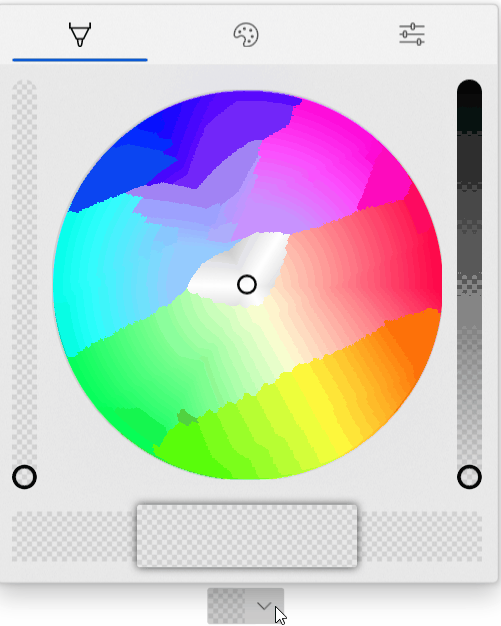
The new properties for this picker are below. Note that these are purposely name 'CustomPalette...' instead of 'Palette...' as multiple palettes may be supported in the future (windows colors, recent colors, custom palette all in different sections visible at the same time).
```csharp
/// <summary>
/// Gets the list of custom palette colors.
/// </summary>
public ObservableCollection<Windows.UI.Color> CustomPaletteColors { get; }
/// <summary>
/// Gets or sets the number of colors in each row (section) of the custom color palette.
/// A section is the number of columns within an entire row in the palette.
/// Within a standard palette, rows are shades and columns are unique colors.
/// </summary>
public int CustomPaletteColumns { get; set; }
/// <summary>
/// Gets or sets a custom IColorPalette .
/// This will automatically set <see cref="CustomPaletteColors"/> and <see cref="CustomPaletteSectionCount"/>
/// overwriting any existing values.
/// </summary>
public IColorPalette CustomPalette { get; set; }
/// <summary>
/// Gets or sets a value indicating whether the color palette is visible.
/// </summary>
public bool IsColorPaletteVisible { get; set; }
```
Making all of this easier is a new IColorPalette interface. Any class can implement this interface to provide a custom color palette such as windows colors (the default), RYB colors, Flat UI colors, etc. Colors could also be algorithmically generated.
```csharp
/// <summary>
/// Interface to define a color palette.
/// </summary>
public interface IColorPalette
{
/// <summary>
/// Gets the total number of colors in this palette.
/// A color is not necessarily a single value and may be composed of several shades.
/// </summary>
int ColorCount { get; }
/// <summary>
/// Gets the total number of shades for each color in this palette.
/// Shades are usually a variation of the color lightening or darkening it.
/// </summary>
int ShadeCount { get; }
/// <summary>
/// Gets a color in the palette by index.
/// </summary>
/// <param name="colorIndex">The index of the color in the palette.
/// The index must be between zero and <see cref="ColorCount"/>.</param>
/// <param name="shadeIndex">The index of the color shade in the palette.
/// The index must be between zero and <see cref="ShadeCount"/>.</param>
/// <returns>The color at the specified index or an exception.</returns>
Color GetColor(int colorIndex, int shadeIndex);
}
```
## PR Checklist
Please check if your PR fulfills the following requirements:
- [x] Tested code with current [supported SDKs](../readme.md#supported)
- [ ] Pull Request has been submitted to the documentation repository [instructions](..\contributing.md#docs). Link: <!-- docs PR link -->
- [x] Sample in sample app has been added / updated (for bug fixes / features)
- [x] Icon has been created (if new sample) following the [Thumbnail Style Guide and templates](https://github.com/windows-toolkit/WindowsCommunityToolkit-design-assets)
- [ ] Tests for the changes have been added (for bug fixes / features) (if applicable)
- [x] Header has been added to all new source files (run *build/UpdateHeaders.bat*)
- [x] Contains **NO** breaking changes
## Other information
**Control**
* [x] Ensure the name ColorPickerButton is accepted
* [x] Rename ColorPickerSlider to ColorPickerButtonSlider. ColorPickerSlider is already used by WinUI.
* [x] Implement all accessibility functionality. Check tab ordering.
* [x] This control originally used NumberBoxes for channel numerical input. Complete porting this functionality over to TextBoxes as the Toolkit does not have a dependency on WinUI.
* [x] Support all visual state groups in the original ColorPicker. Connect all functionality
* [x] Test changing all ColorPicker properties. Determine which ones make sense to ignore
* [x] Trim displayed hex if alpha is not enabled
* [x] ~~Switch to radio buttons to switch between RGB/HSV representation. A ComboBox within a Flyout is generally bad practice and is also limited by some bugs in UWP (#1467).~~
* [x] Switch to ToggleButton instead of RadioButton for RGB/HSV representation. Radio buttons don't work in flyouts and controls well due to UWP bugs. ToggleButtons may also visually look better. The design follows a 'segmented control' concept discussed here: https://github.com/microsoft/microsoft-ui-xaml/issues/2310
* [x] Switch the default color palette to be the windows colors (if possible)
* [x] Align formatting to the toolkit conventions
* [x] Handle CornerRadius in the template. Conditional XAML? Check how other controls do this.
* [x] Rename 'CustomPaletteSectionCount' to ~~'CustomPaletteWrapCount'~~ or 'CustomPaletteColumns' like UniformGrid
* [x] Update selected palette color data template to provide correct contrast of the border with the selected color ~~to match with windows settings app (check mark overlay)~~ The check mark is not included as the size of swatches in the palette could be smaller than the check mark itself.
* [x] Rename 'CustomPaletteColumns' to 'CustomPaletteColumnCount'
* [ ] Treat negative/zero numbers in CustomPaletteColumnCount as 'auto' and automatically calculate a good column count to keep rows/columns even. Set the default of this property to 0 'auto'.
* [x] Improve spacing above/below channel sliders -- try 24px (2 x 12px)
* [ ] Move localizable strings into resources
* [x] ~Do not show the color (set the preview border visibility to collapsed) while the control is initializing. This prevents the default color from briefly appearing during initial load. Instead, the background will just show through.~
* [x] Do not change the saturation and value in the hue slider background. Keep SV at maximum. This allows the hue to always be clear to the user and is in line with other implementations (like in Inkscape).
* [x] Rename `WindowsColorPalette` to `FluentColorPalette`
* [x] Show palette color display name in a tooltip
* [x] Use the WinUI algorithm for light/dark color switching
* [x] Each tab should have independent visibility using IsColorPaletteVisible (new), IsColorSpectrumVisible (existing) and IsColorChannelTextInputVisible (repurposed to mean the entire tab). The tabs still visible should take up available space remaining. With only one tab visible the tab header should disappear.
**Code Review**
1. [x] The mini-palette and color preview will be all one color when the selected color is black or white. The calculated accent colors will not change and pressing them will not change the selected color.
* Requires feedback from Microsoft on how the accent colors are calculated in Windows
* It is possible to specially handle when the color is as max/min value (black or white) at least the two accent colors to the left/right could be different shades of gray.
1. [x] The mini-palette accent colors are destructive. Pressing back and forward by 1 will not restore the original color.
* Again, requires feedback from Microsoft on how the accent colors are calculated in Windows.
* Due to perceptual adjustments it's likely this will always be destructive.
1. [x] The third dimension slider in the spectrum tab will vary the other dimensions in the spectrum. This results in the spectrum cursor jumping around as the slider value changes. This might be related to HSV/RGB conversion but needs to be investigated.
1. [x] Adjust the design of the sample app page to move the ColorPickerButton closer to the description.
1. [x] Check if a ColorToDisplayName converter already exists. Consider adding control specific converters to their own sub-namespace.
1. [x] The ColorPickerButton will derive from DropDownButton and have a ColorPicker property. Dependent on #3440
1. [x] The ColorPicker will have the same new style as the ColorPickerButton flyout. While it's a new concept to have tabs in a panel-type control, this is considered acceptable.
1. [x] Merge @michael-hawker branch into this one when given the thumb's up. Both controls will be in the same directory.
1. [x] Remove conditional XAML for corner radius after WinUI 3.0. Conditional XAML doesn't work correctly in resource dictionaries and with setters. See https://github.com/microsoft/microsoft-ui-xaml/issues/2556, fixed with #3440
1. [ ] All slider rendering will be broken out and put into a new ColorPickerSlider. This should simplify code-behind and the default template.
1. [x] The Pivot tab width and show/hide of tabs will be controlled in code-behind. use of the pivot itself is somewhat dependent on issues with touch. The pivot, or switching to a new control, will not hold the release.
1. [ ] Sliders should vary only 1-channel and keep the other channels at maximum. This ensures the gradient always makes sense and never becomes fully black/white/transparent. This could also be made a configuration of the ColorPickerSlider control. Edit: This does need to be configurable as it's only required in HSV mode.
1. [ ] Add color history palette to the sample app
**Other**
* [x] Complete integration with the sample app. Add XAML to dynamically change the control.
* [ ] Complete documentation of the control
**Future**
These changes should be considered for future versions of the control. They are recorded here for lack of a better location.
* [ ] The mini selected color palette may follow the Windows accent color lighter/darker shades algorithm. However, the implementation of this algorithm is currently unknown. This algorithm may be in the XAML fluent theme editor: https://github.com/microsoft/fluent-xaml-theme-editor
* [ ] Switch back to NumberBox for all color channel numerical input. This will require WinUI 3.0 and the corresponding release of the toolkit. When https://github.com/microsoft/microsoft-ui-xaml/issues/2757 is implemented, also use NumberBox for hex color input.
* [ ] ~Remove the custom 'ColorPickerButtonSlider' after switch to WinUI 3.0. This assumes there is no additional control-specific code at that point. (It currently exists just to work-around UWP bugs).~ This should be turned into it's own full primitive control.
* [ ] Update ColorSpectrum_GotFocus event handler (instructions are within code) after/if ColorSpectrum bugs are fixed (likely after WinUI 3.0
* [ ] Use Color.IsEmpty if https://github.com/microsoft/microsoft-ui-xaml/issues/2826 is implemented
* [ ] ~Ensure PivotItems are properly removed once/if https://github.com/microsoft/microsoft-ui-xaml/issues/2952 is closed.~
<!-- 🚨 Please Do Not skip any instructions and information mentioned below as they are all required and essential to evaluate and test the PR. By fulfilling all the required information you will be able to reduce the volume of questions and most likely help merge the PR faster 🚨 -->
## Follow up for #3346 and #3455
<!-- Add the relevant issue number after the "#" mentioned above (for ex: Fixes#1234) which will automatically close the issue once the PR is merged. -->
<!-- Add a brief overview here of the feature/bug & fix. -->
## PR Type
What kind of change does this PR introduce?
<!-- Please uncomment one or more that apply to this PR. -->
<!-- - Bugfix -->
- Feature
<!-- - Code style update (formatting) -->
<!-- - Refactoring (no functional changes, no api changes) -->
<!-- - Build or CI related changes -->
<!-- - Documentation content changes -->
<!-- - Sample app changes -->
<!-- - Other... Please describe: -->
## What is the current behavior?
<!-- Please describe the current behavior that you are modifying, or link to a relevant issue. -->
The `ThrowHelper` class exposes static throw-helper APIs that mirror the existing constructor for all the supported exception types. In the previous PRs though I had missed the parameterless constructors, so there were no APIs for that in the `ThrowHelper` class.
## What is the new behavior?
<!-- Describe how was this issue resolved or changed? -->
`ThrowHelper` now exposes a parameterless overload for all the supported exception types that are exposed.
## PR Checklist
Please check if your PR fulfills the following requirements:
- [X] Tested code with current [supported SDKs](../readme.md#supported)
- [ ] ~~Pull Request has been submitted to the documentation repository [instructions](..\contributing.md#docs). Link: <!-- docs PR link -->~~
- [ ] ~~Sample in sample app has been added / updated (for bug fixes / features)~~
- [ ] ~~Icon has been created (if new sample) following the [Thumbnail Style Guide and templates](https://github.com/windows-toolkit/WindowsCommunityToolkit-design-assets)~~
- [X] Tests for the changes have been added (for bug fixes / features) (if applicable)
- [X] Header has been added to all new source files (run *build/UpdateHeaders.bat*)
- [X] Contains **NO** breaking changes